Algorithm-driven team building
Programming Snapshot – Go Algorithms
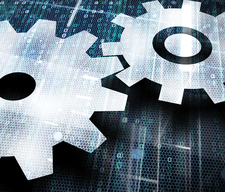
© Lead Image © bowie15, 123RF.com
Instead of the coach determining the team lineup, an algorithm selects the players based on their strengths for Mike Schilli's amateur soccer team.
At the weekly pickup soccer game in my adopted hometown of San Francisco, with 16 players signed up for an eight-on-eight match, there is always the question of who is going to compete against whom on match day. Preparing the team sheet is usually something taken care of by experienced players, but it is time-consuming and it leads to endless discussions about whether the eight players on one side are simply too skilled for the other eight players, leading to a lopsided game that is no fun to play. To resolve this, I've developed an algorithm to generate the team lineup. This allows me to take an interesting excursion into the world of combinatorics and explore the development of suitable algorithms in this month's column.
How many ways are there to assign 16 players to two teams of eight players each? If one team is fixed, the opponent's team is automatically derived from the remaining participants; this means that the result is written in combinatorics style as "8 over 16," which you compute as
(16*15*...*10*9) / (8*7*...*2*1)
[...]
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
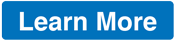
News
-
Akamai Will Host kernel.org
The organization dedicated to cloud-based solutions has agreed to host kernel.org to deliver long-term stability for the development team.
-
Linux Kernel 6.14 Released
The latest Linux kernel has arrived with extra Rust support and more.
-
EndeavorOS Mercury Neo Available
A new release from the EndeavorOS team ships with Plasma 6.3 and other goodies.
-
Fedora 42 Beta Has Arrived
The Fedora Project has announced the availability of the first beta release for version 42 of the open-source distribution.
-
Dash to Panel Maintainer Quits
Charles Gagnon has stepped away as maintainer of the popular Dash to Panel Gnome extension.
-
CIQ Releases Security-Hardened Version of Rocky Linux
If you're looking for an enterprise-grade Linux distribution that is hardened for business use, there's a new version of Rocky Linux that's sure to make you and your company happy.
-
Gnome’s Dash to Panel Extension Gets a Massive Update
If you're a fan of the Gnome Dash to Panel extension, you'll be thrilled to hear that a new version has been released with a dock mode.
-
Blender App Makes it to the Big Screen
The animated film "Flow" won the Oscar for Best Animated Feature at the 97th Academy Awards held on March 2, 2025 and Blender was a part of it.
-
Linux Mint Retools the Cinnamon App Launcher
The developers of Linux Mint are working on an improved Cinnamon App Launcher with a better, more accessible UI.
-
New Linux Tool for Security Issues
Seal Security is launching a new solution to automate fixing Linux vulnerabilities.