Network diagnostics with Go
Dr. Wireless
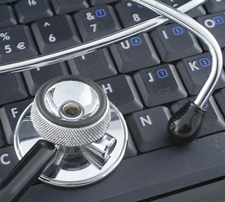
© Lead Image © Ewa Walicka, Fotolia.com
Why is the WiFi not working? Instead of always typing the same steps to diagnose the problem, Mike Schilli writes a tool in Go that puts the wireless network through its paces and helps isolate the cause.
Imagine you've just arrived at your vacation resort, and the WiFi isn't working. Is the router's DHCP server failing to assign an IP address to your laptop? Is it DNS? Or is it just that the throughput is so poor that everything seems to be stalling?
You can diagnose all of these issues by running various command-line tools, but it is tedious and annoying to have to repeat the procedure every time. How about a tool that repeatedly runs these steps at regular intervals, visualizes the results, and hopefully zeroes in on the root cause?
I will use the tview [1] library from GitHub as the terminal user interface (UI) for my wifi
diagnostic tool. After all, some well-known projects, such as Kubernetes, also use it for their command-line tools. With just a few lines of code, tview switches the current terminal to raw mode and displays simple graphical elements such as tables or forms in a retro white on black background style0 (Figure 1). It accepts keyboard input in raw mode, and applications can use it to control actions on the interface.
[...]
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
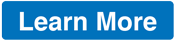
News
-
OSJH and LPI Release 2024 Open Source Pros Job Survey Results
See what open source professionals look for in a new role.
-
Proton 9.0-1 Released to Improve Gaming with Steam
The latest release of Proton 9 adds several improvements and fixes an issue that has been problematic for Linux users.
-
So Long Neofetch and Thanks for the Info
Today is a day that every Linux user who enjoys bragging about their system(s) will mourn, as Neofetch has come to an end.
-
Ubuntu 24.04 Comes with a “Flaw"
If you're thinking you might want to upgrade from your current Ubuntu release to the latest, there's something you might want to consider before doing so.
-
Canonical Releases Ubuntu 24.04
After a brief pause because of the XZ vulnerability, Ubuntu 24.04 is now available for install.
-
Linux Servers Targeted by Akira Ransomware
A group of bad actors who have already extorted $42 million have their sights set on the Linux platform.
-
TUXEDO Computers Unveils Linux Laptop Featuring AMD Ryzen CPU
This latest release is the first laptop to include the new CPU from Ryzen and Linux preinstalled.
-
XZ Gets the All-Clear
The back door xz vulnerability has been officially reverted for Fedora 40 and versions 38 and 39 were never affected.
-
Canonical Collaborates with Qualcomm on New Venture
This new joint effort is geared toward bringing Ubuntu and Ubuntu Core to Qualcomm-powered devices.
-
Kodi 21.0 Open-Source Entertainment Hub Released
After a year of development, the award-winning Kodi cross-platform, media center software is now available with many new additions and improvements.