Manipulating Binary Data with Bash
Bit Trip
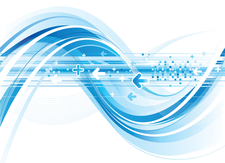
© Lead Image © Yewkeo, 123RF.com
Bash is known for admin utilities and text manipulation tools, but the venerable command shell included with most Linux systems also has some powerful commands for manipulating binary data.
One of the most versatile scripting environments available on Linux is the Bash shell. The core functionality of Bash includes many mechanisms for tasks such as string processing, mathematical computation, data I/O, and process management. When you couple Bash with the countless command-line utilities available for everything from image processing to virtual machine (VM) management, you have a very powerful scripting platform.
One thing that Bash is not generally known for is its ability to process data at the bit level; however, the Bash shell contains several powerful commands that allow you to manipulate and edit binary data. This article describes some of these binary commands and shows them at work in some practical situations.
Viewing and Converting Data
Two tools you can use to represent data in hexadecimal and binary format are hexdump
and xxd
. The hexdump
utility provides many options for outputting hexadecimal data. Although xxd
lacks some of the options available with hexdump
, it does have one key feature hexdump
lacks: In addition to letting you output hexadecimal values, xxd
also has the ability to convert a hexadecimal string into binary data. The following command:
command> echo -n "hello" | xxd -p output> 68656c6c6f
outputs the binary values of the ASCII string "hello" as an ASCII string of hexadecimal values (refer to Table 1).
Table 1
ASCII Lowercase Alphabet
Char | Decimal | Hexadecimal |
---|---|---|
a |
97 |
61 |
b |
98 |
62 |
c |
99 |
63 |
d |
100 |
64 |
e |
101 |
65 |
f |
102 |
66 |
g |
103 |
67 |
h |
104 |
68 |
i |
105 |
69 |
j |
106 |
6A |
k |
107 |
6B |
l |
108 |
6C |
m |
109 |
6D |
n |
110 |
6E |
o |
111 |
6F |
p |
112 |
70 |
q |
113 |
71 |
r |
114 |
72 |
s |
115 |
73 |
t |
116 |
74 |
u |
117 |
75 |
v |
118 |
76 |
w |
119 |
77 |
x |
120 |
78 |
y |
121 |
79 |
z |
122 |
7A |
In the following command:
command> echo -n "6162" | xxd -p -r output> ab
The hexadecimal string "6162" is converted into what the xxd
man page calls a "mail-safe ASCII representation" of the binary data. Because hexadecimal 61 and 62 correspond to ASCII characters "a" and "b," respectively, the binary data is expressed in the form of the string "ab."
Bash makes it easy to build these commands into functions. The following functions, pack
and unpack
(named because of their similarity to the PHP pack
function) use the preceding commands to convert a hexadecimal string to binary and convert binary to hex.
pack() { echo -n "$1" | xxd -p -r } unpack() { echo -n "$1" | xxd -p }
All the examples so far have used the -p
switch to specify that the command will use plain hexdump style, representing each 8-bit byte as a two-digit hexadecimal number. To output data as a binary string (containing 1s and 0s), you need to use different switches. The following function returns a binary string representation of binary data, along with a sample command and output:
tobin() { echo -n "$1" | xxd -b -g0 | awk '{ printf("%s",$2) }' } command> tobin ab output> 0110000101100010
The two-character string "ab" is converted into a binary string containing two 8-bit values: 01100001 and 01100010 (97 and 98), corresponding to the base 10 values for characters "a" and "b."
Transforming Binary Data
Bash also offers some commands for transforming binary data using mathematical and logical operations. This process is call arithmetic expansion. In the following example:
command> echo $(( 4*5 )) output> 20
the output of the echo
command is the value calculated by the statement enclosed in $((
and ))
.
Another tool for performing mathematical operations is bc
. bc
is a command-line calculator that can read statements from standard input. For example:
command> echo "4*5" | bc output> 20
Note that the preceding operation is performed in base 10. bc
does not provide native functionality for arithematic operations on hexadecimal numbers, so you need to convert the numbers to base 10 and then perform the operation. For instance, if you want to perform simple addition and subtraction operations against hexadecimal numbers, use a function like the following:
hexadd() { echo "obase=16;ibase=A;$((16#$1))+$2" | bc } command> hexadd A 2 output> C
The hexadd
function provides the ability to add or subtract (add a negative number) to a valid hexadecimal number in a single command. The function pipes a string of commands into bc
. The first command, obase=16
, sets the base in which data will be output (in this case, hexadecimal or base 16). The second command, ibase=A
, sets the base used to read input data. The input base is set up A
, which corresponds to base 10. The third and final command is an addition statement comprised of an arithmetic expansion and the second function argument. The arithmetic expansion uses the #
operator to convert the number following it from the base specified before the #
to base 10. In the command, the hexadecimal value for "A" is converted to decimal 10 using arithmetic expansion, added to 2 using bc
, and then converted back into hexadecimal using bc
.
You can also perform bitwise operations on the data, including AND, OR, XOR, and shift. The AND operation (using the &
operator) returns the bits shared between the two numbers. The OR operation (using the |
operator) returns all bits used by either of the two numbers. The XOR operation (using the ^ operator) returns all bits that are unique to one of the numbers.
In Listing 1, the first command performs a binary AND on 3 (or 0011 in binary) and 6 (or 0110 in binary). Because the only shared bit is the second bit, 2 (or 0010 in binary), the output for the command is 2. The second command performs an OR operation against the same two numbers: 3 and 6. Between the two numbers, the lower three bits are used, so 7 (or 0111 in binary) is returned. In the third example an XOR is performed against the same two numbers. The second and fourth bits are the same between the two numbers, however, the third and first bit are different, therefore 5 (or 0101 in binary), is returned.
Listing 1
Bitwise Operations
01 logicand() { 02 echo $(($1&$2)) 03 } 04 05 logicor() { 06 echo $(($1|$2)) 07 } 08 09 logicxor() { 10 echo $(($1^$2)) 11 } 12 13 command> logicand 3 6 14 output> 2 15 command> logicor 3 6 16 output> 7 17 command> logicxor 3 6 18 output> 5
The other bitwise operations is the shift operation, which shifts the bits in one direction or another (right or left). Listing 2 shows functions for shifting the bits shifting right (using the >>
operator) and left (using the <<
operator).
Listing 2
Shifting Bits
01 shiftr() { 02 echo $(($1>>$2)) 03 } 04 05 shiftl() { 06 echo $(($1<<$2)) 07 } 08 09 command> shiftl 4 2 10 output> 16 11 command> shiftr 16 4 12 output> 1
The first command in Listing 2 shifts the bits in 4 (0100) left by two spaces, returning a value of 16 (10000). The second command shifts the bits in 16 (10000) to the right four spaces, returning a value of 1 (0001). As you might have noticed, for each bit space shifted to the left, the number is multiplied by 2, and for each bit space shifted to the right, the number is divided by 2.
Examples
Switching from binary to hexadecimal, and moving bits around to change an A into a C, is certainly interesting, but does this capability have any uses in the real world? The following examples offer some hints for how you could use these tools in practical ways.
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
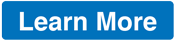
News
-
Gnome OS Transitioning Toward a General-Purpose Distro
If you're looking for the perfectly vanilla take on the Gnome desktop, Gnome OS might be for you.
-
Fedora 41 Released with New Features
If you're a Fedora fan or just looking for a Linux distribution to help you migrate from Windows, Fedora 41 might be just the ticket.
-
AlmaLinux OS Kitten 10 Gives Power Users a Sneak Preview
If you're looking to kick the tires of AlmaLinux's upstream version, the developers have a purrfect solution.
-
Gnome 47.1 Released with a Few Fixes
The latest release of the Gnome desktop is all about fixing a few nagging issues and not about bringing new features into the mix.
-
System76 Unveils an Ampere-Powered Thelio Desktop
If you're looking for a new desktop system for developing autonomous driving and software-defined vehicle solutions. System76 has you covered.
-
VirtualBox 7.1.4 Includes Initial Support for Linux kernel 6.12
The latest version of VirtualBox has arrived and it not only adds initial support for kernel 6.12 but another feature that will make using the virtual machine tool much easier.
-
New Slimbook EVO with Raw AMD Ryzen Power
If you're looking for serious power in a 14" ultrabook that is powered by Linux, Slimbook has just the thing for you.
-
The Gnome Foundation Struggling to Stay Afloat
The foundation behind the Gnome desktop environment is having to go through some serious belt-tightening due to continued financial problems.
-
Thousands of Linux Servers Infected with Stealth Malware Since 2021
Perfctl is capable of remaining undetected, which makes it dangerous and hard to mitigate.
-
Halcyon Creates Anti-Ransomware Protection for Linux
As more Linux systems are targeted by ransomware, Halcyon is stepping up its protection.